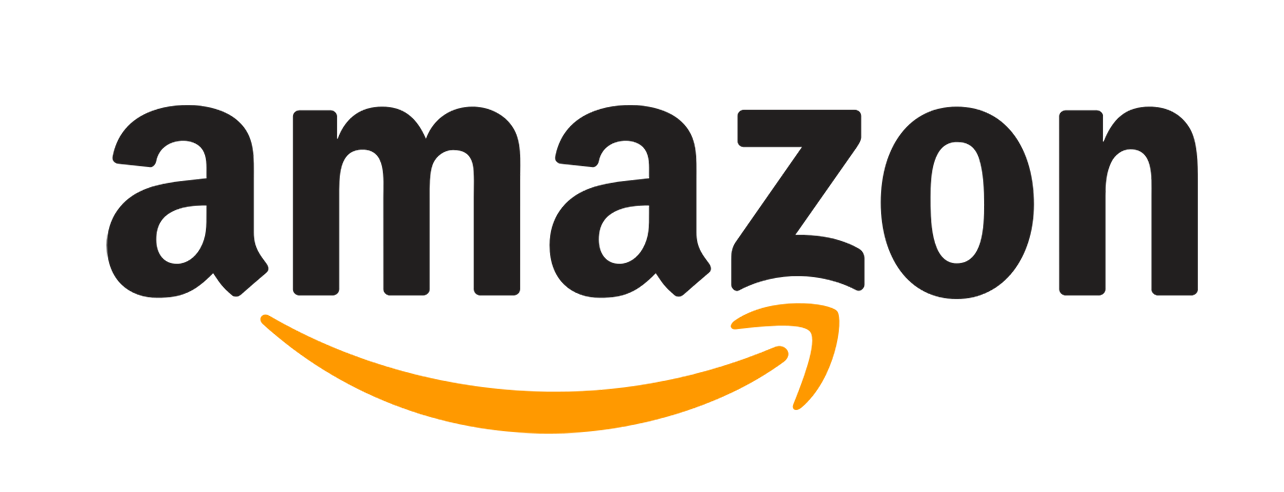
Mission: We strive to offer our customers the lowest possible prices, the best available selection, and the utmost convenience
Vision: To be Earth"s most customer-centric company, where customers can find and discover anything they might want to buy online.
Out of the Amazon Leadership Principles, which principle do you feel like is your greatest strength?
Tell me about a time you went above and beyond for a customer.
Tell me about a time you solved a problem for a customer.
Tell me about how you understand your customer to best meet their needs.
Tell me about a time when you experienced a major failure.
Tell me about a time when you had to make a hard choice.
Tell me about a time when you had to think long-term.
Tell me about a time when you had to leave a task unfinished.
Tell me about a time when you invented something.
Tell me about a time when you gave a simple solution to a complex problem.
Tell me about a time when you had to work with incomplete data or information.
Tell me about a time when you solved a problem through just superior knowledge or observation.
Tell me about something you’re learning now.
Tell me about a time when there was a dispute on your team.
Tell me about feedback you’ve received recently and how it affected you.
Give me two examples of when you did more than what was required in any job experience.
Tell me about a time when you had to decide between many different choices.
Tell me about a time when you took a calculated risk.
Tell me about a time when you had to work with limited time or resources.
Tell me about how you build trust within a new team.
Tell me about a time you faced conflict.
Tell me about how data informs your decisions.
Tell me about a time when you influenced a change by only asking questions.
Tell me about an unpopular decision of yours.
Tell me about a time you were behind on a hard deadline.
Tell me about your proudest professional achievement.
Stage 1: An online Work Style assessment that determines if you align with their leadership principles and display key skills. Most of the assessment is a simulation of how you’d solve common problems at work, like emails and task prioritization.
Stage 2: A phone screen with a team member based on your resume
Stage 3: A phone screen with a coding component (Machine learning, Algorithms, Python, SQL)
Stage 4: An onsite interview composed of 4-7 sessions. Usually 6+ one-on-one interviews" 5 formal and 1 informal interview over lunch. Each interview tests at least 1 leadership principle as well as problem solving skills. The questions cover SQL, statistics, machine learning, predictive modelling, exploratory analysis, and more.
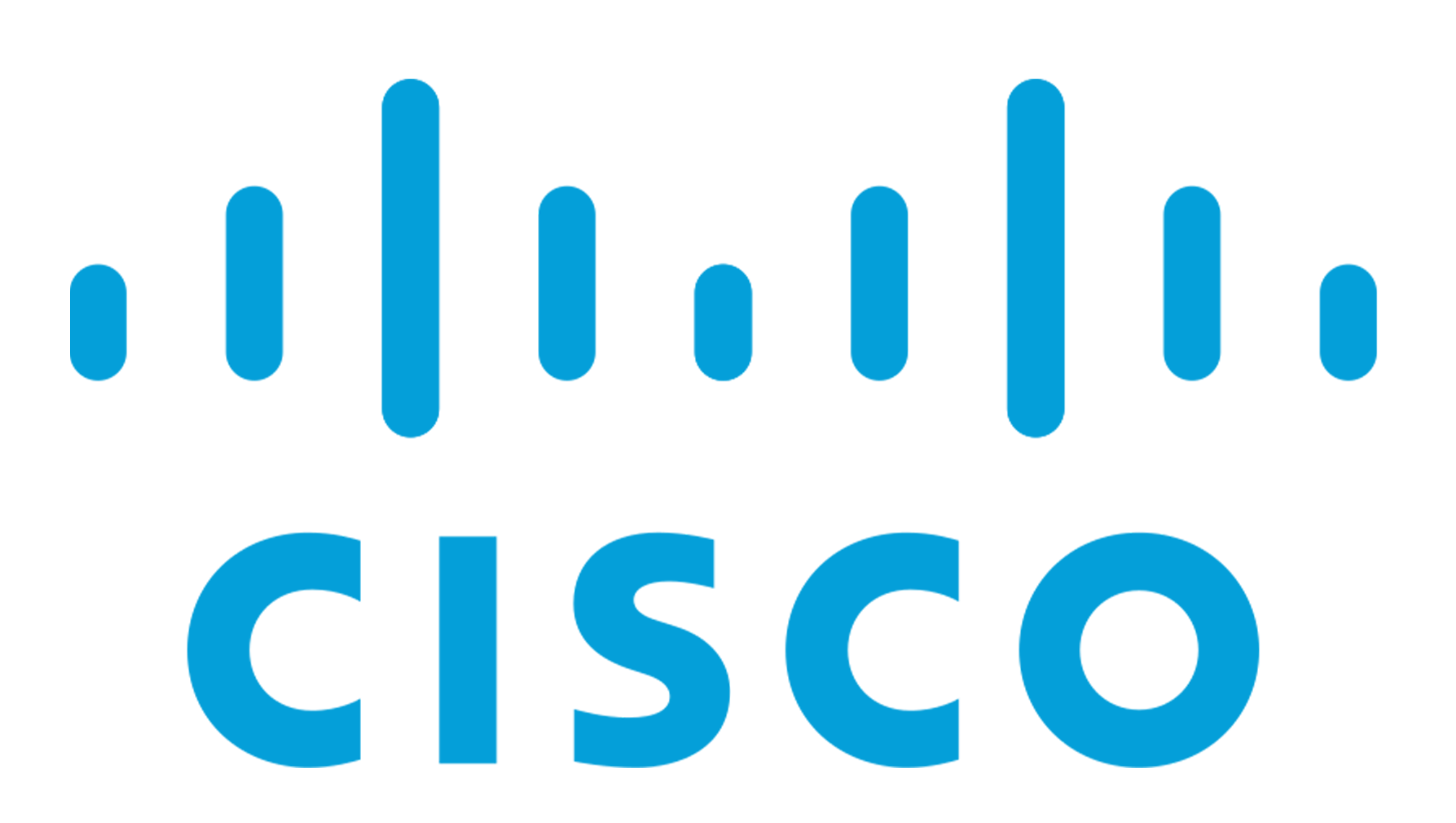

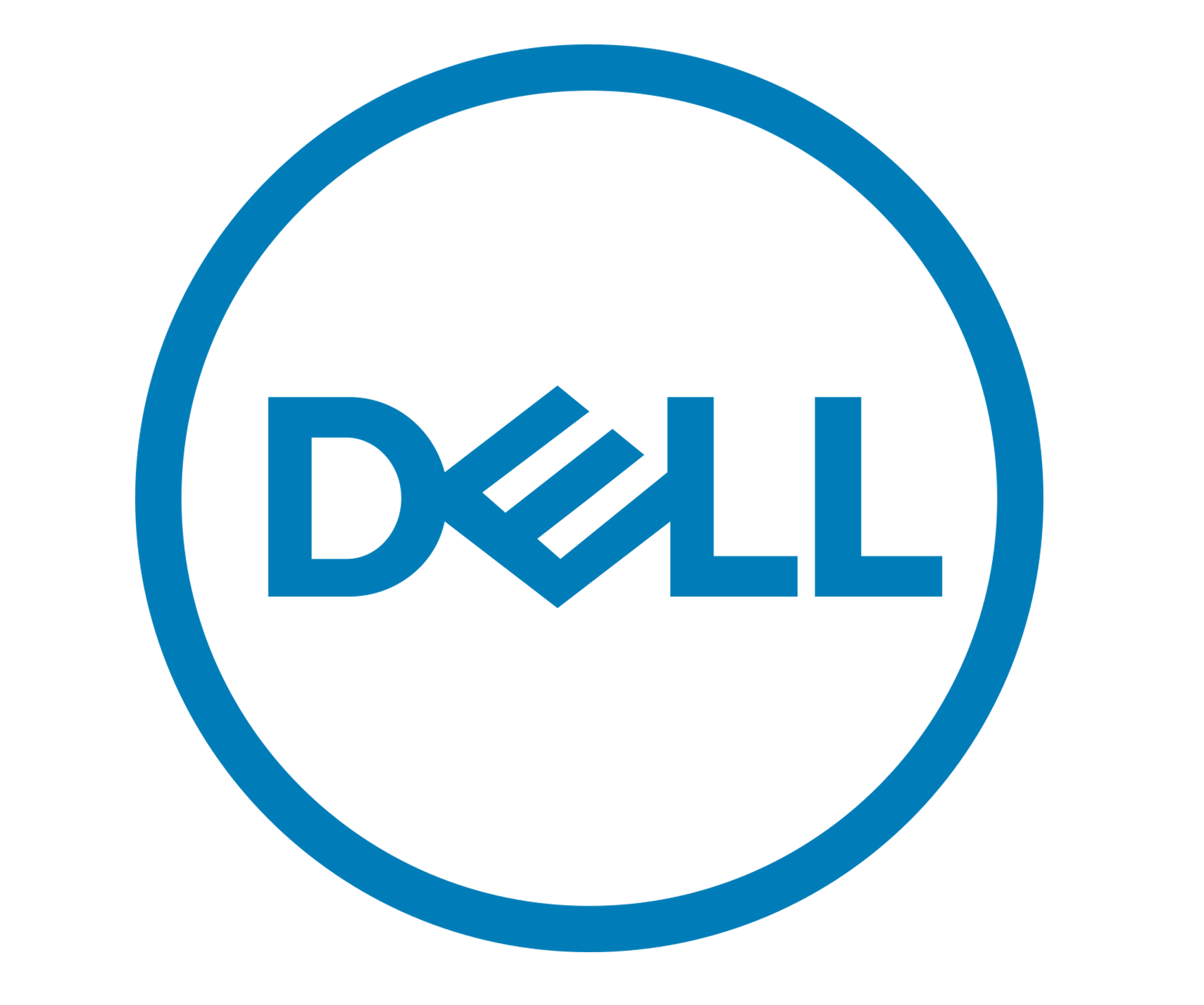
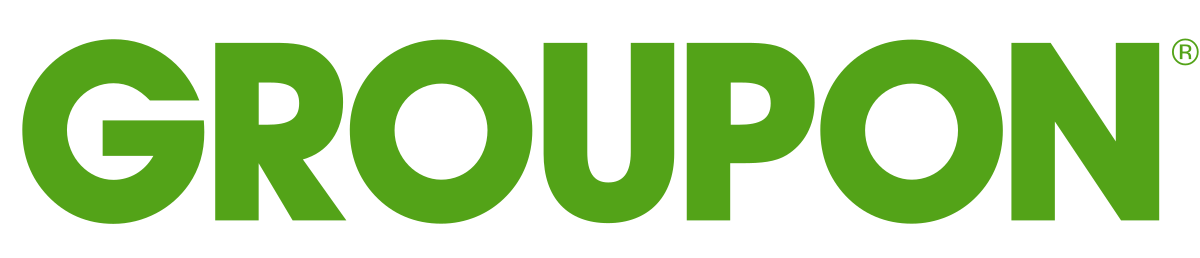
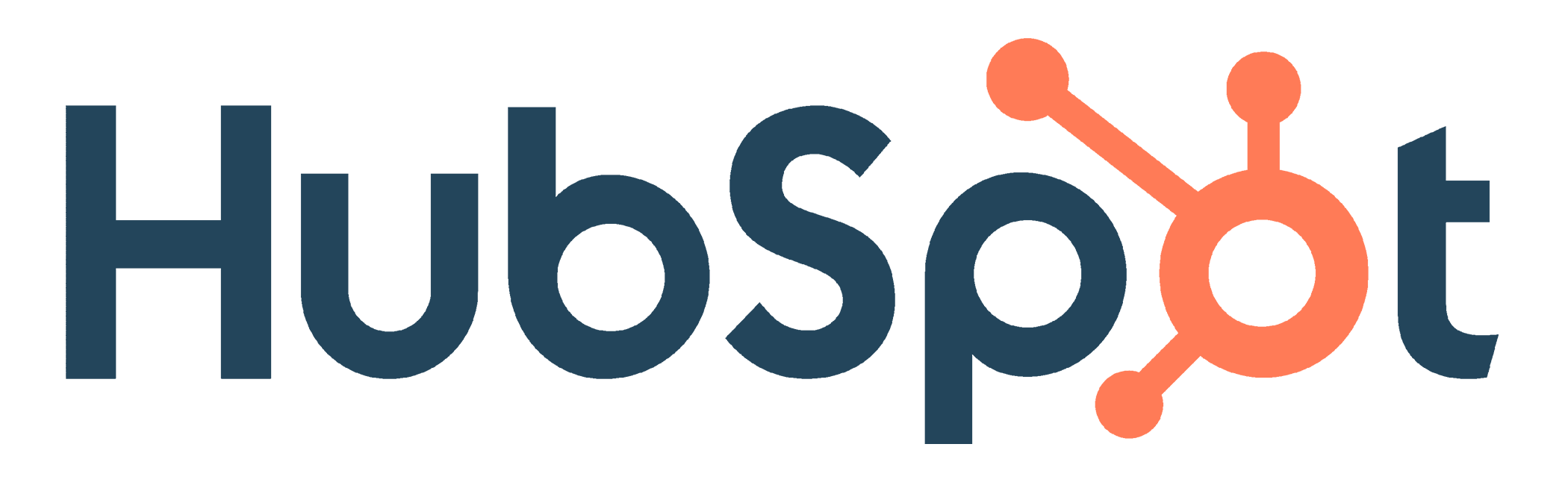

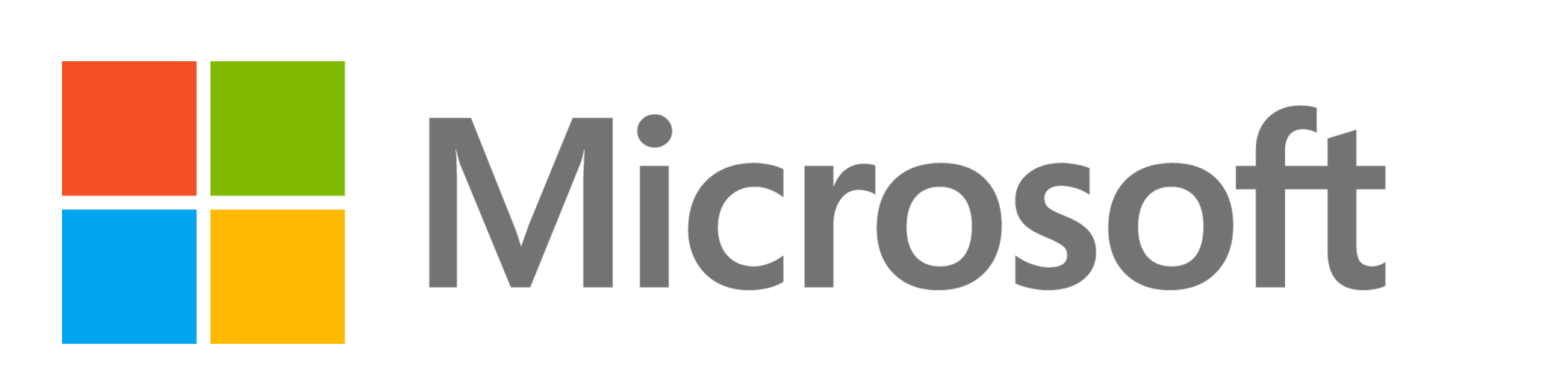
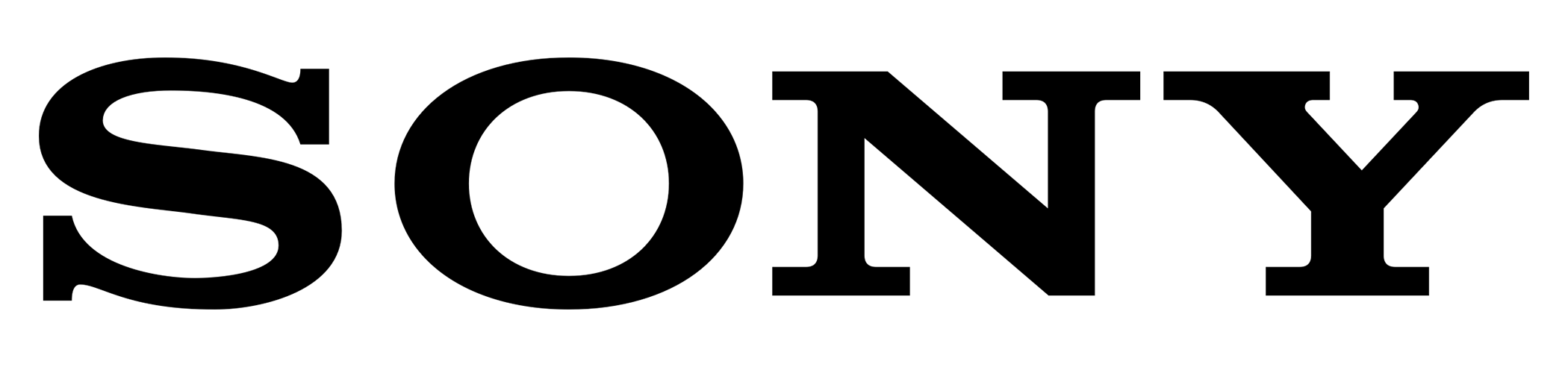
Pathrise is a career accelerator that helps people land their dream jobs. We regularly place our fellows at top companies like Apple, Amazon, and Meta. Our mentors have experience at companies like Apple, giving fellows the inside scoop on interview and company culture in 1-on-1 sessions.
We can’t guarantee you a job at a specific company like Apple. But we do guarantee you a great job–if you don’t accept an offer in 1 year, you pay nothing. Our income share agreement means you only pay with a percentage of your income at your new role.
Mentors work with fellows at every stage in search, helping them build the skills necessary to be the best candidate possible. Fellows in Pathrise usually see a 2-4x increase in application response rates, 1.5-3x increase in interview scores, and 10-20% increase in salary through negotiation.